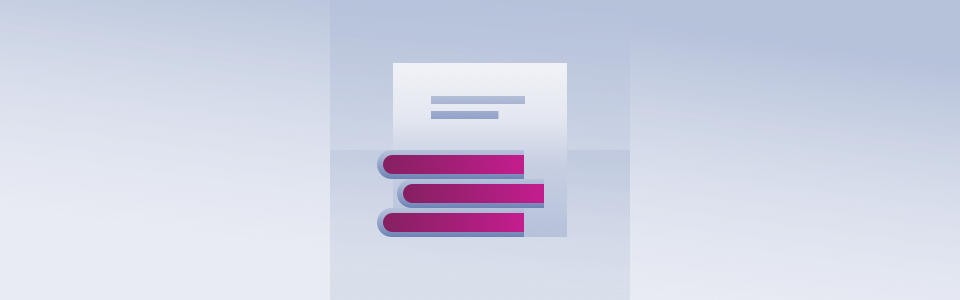
Dynamic Form Fields: Using AJAX to Auto-Update HTML Content Based on User Selection
Have you ever needed to automatically update one form field based on the value of another? For example, selecting a country and having the states/provinces dropdown automatically populate? In this tutorial, we'll walk through how to implement this common functionality using AJAX.
Let's say we have a product selection form where choosing a product should automatically update the price field. Traditionally, this would require a full page reload, but with AJAX, we can make it smooth and seamless.
First, let's create our basic form structure:
<form id="productForm">
<div class="form-group">
<label for="productSelect">Select Product:</label>
<select id="productSelect" name="product">
<option value="">Choose a product...</option>
<option value="1">Premium Widget</option>
<option value="2">Basic Widget</option>
<option value="3">Super Widget</option>
</select>
</div>
<div class="form-group">
<label for="priceField">Price:</label>
<input type="text" id="priceField" name="price" readonly>
</div>
</form>
Here's how we'll handle the AJAX update:
document.addEventListener('DOMContentLoaded', function() {
const productSelect = document.getElementById('productSelect');
const priceField = document.getElementById('priceField');
productSelect.addEventListener('change', function() {
const selectedProduct = this.value;
if (!selectedProduct) {
priceField.value = '';
return;
}
// Make the AJAX request
fetch('/api/get-price', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
productId: selectedProduct
})
})
.then(response => response.json())
.then(data => {
priceField.value = data.price;
})
.catch(error => {
console.error('Error fetching price:', error);
priceField.value = 'Error loading price';
});
});
});
Here's an example of how the server-side handler might look in PHP:
<?php
header('Content-Type: application/json');
// Get the POST data
$data = json_decode(file_get_contents('php://input'), true);
$productId = $data['productId'];
// In a real application, you would fetch this from a database
$prices = [
1 => 99.99,
2 => 49.99,
3 => 149.99
];
$response = [
'price' => isset($prices[$productId]) ? $prices[$productId] : null
];
echo json_encode($response);
?>
We've implemented proper error handling both on the client and server side. The price field shows loading states and error messages when appropriate. The form still works without JavaScript, though it would require a page reload. The server validates the product ID before returning a price.
When implementing this feature, keep these security points in mind:
- Always validate user input on both client and server side
- Use CSRF tokens for POST requests
- Sanitize data before displaying it back to the user
- Implement proper access controls on the API endpoint
To make your AJAX updates even more efficient:
- Debounce the AJAX requests if the field can change rapidly
- Cache responses for frequently requested values
- Consider using a loading indicator for slower connections
The code above uses the Fetch API, which works in all modern browsers. For older browsers, you might want to use a polyfill or switch to XMLHttpRequest:
function makeRequest(url, data, callback) {
const xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.onreadystatechange = function() {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
callback(null, JSON.parse(xhr.responseText));
} else {
callback(new Error('Request failed'));
}
}
};
xhr.send(JSON.stringify(data));
}
Dynamically updating form fields with AJAX creates a smoother user experience and reduces server load by eliminating unnecessary page reloads. By following the patterns and best practices outlined above, you can implement this functionality in a robust and maintainable way.
Remember to test thoroughly across different browsers and network conditions, and always prioritize the user experience by providing appropriate feedback during loading and error states.
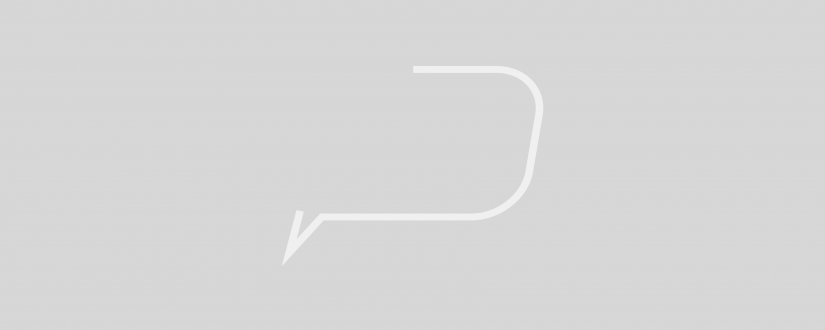