Securing Password Storage in Drupal 7: Best Practices Guide
Password security remains a critical concern in web development, especially given the increasing frequency of cyber attacks. This guide explains how to implement secure password storage in Drupal 7 using modern PHP password hashing techniques.
Understanding Password Security Fundamentals
Secure password storage relies on proper cryptographic hashing rather than simple encryption or plain text storage. Drupal 7's default password handling is quite robust, but understanding how to implement additional security measures can be valuable for custom implementations or specific requirements.
Implementing Secure Password Storage
Using the Modern Password Hashing API
PHP's password hashing API provides a standardized, secure approach to password management. For Drupal 7 sites running on PHP versions 5.3.7 through 5.5.0, you'll need to install the password_compat library:
composer require ircmaxell/password_compat
This library provides four essential functions for password management:
// Hash a new password for storage
$hashedPassword = password_hash(
$plainTextPassword,
PASSWORD_DEFAULT,
['cost' => 12]
);
// Verify a password against a stored hash
$isValid = password_verify($inputPassword, $storedHash);
// Example implementation
if ($isValid) {
// Password is correct - proceed with authentication
drupal_set_message('Authentication successful');
} else {
// Invalid password
drupal_set_message('Invalid credentials', 'error');
}
// Check if password needs rehashing (e.g., if security parameters have changed)
if (password_needs_rehash($storedHash, PASSWORD_DEFAULT, ['cost' => 12])) {
// Store the new hash
$newHash = password_hash($plainTextPassword, PASSWORD_DEFAULT, ['cost' => 12]);
// Update the stored hash in your database
}
// Get information about the hash
$hashInfo = password_get_info($storedHash);
Integration with Drupal 7
To implement this in your Drupal 7 site, you might create a custom module:
<?php
/**
* Implements hook_user_presave().
* Ensures passwords are properly hashed before saving.
*/
function mymodule_user_presave(&$edit, $account, $category) {
if (isset($edit['pass'])) {
$edit['pass'] = password_hash(
$edit['pass'],
PASSWORD_DEFAULT,
['cost' => 12]
);
}
}
/**
* Custom password verification function.
*/
function mymodule_verify_password($password, $hash) {
return password_verify($password, $hash);
}
Security Considerations
1. Cost Factor: The 'cost' parameter (set to 12 in our examples) determines the computational complexity of the hash. Higher values increase security but also increase processing time. Adjust based on your server capabilities.
2. Hash Storage: Always store the complete hash string, which includes the algorithm, cost, and salt information.
3. Password Validation: Implement proper password complexity requirements:
function mymodule_validate_password_strength($password) {
$errors = array();
if (strlen($password) < 8) {
$errors[] = t('Password must be at least 8 characters long.');
}
if (!preg_match('/[A-Z]/', $password)) {
$errors[] = t('Password must contain at least one uppercase letter.');
}
// Add additional validation as needed
return $errors;
}
Best Practices
1. Never store plain-text passwords
2. Use strong salting (automatically handled by password_hash)
3. Implement password aging policies where appropriate
4. Regular security audits of password-related code
5. Keep PHP and all security-related libraries updated
Error Handling
Always implement proper error handling when dealing with passwords:
try {
$hashedPassword = password_hash($password, PASSWORD_DEFAULT, ['cost' => 12]);
if ($hashedPassword === false) {
watchdog('security', 'Password hashing failed', array(), WATCHDOG_ERROR);
throw new Exception('Password processing error');
}
} catch (Exception $e) {
// Handle the error appropriately
drupal_set_message(t('An error occurred during password processing.'), 'error');
}
```
By following these guidelines and implementing proper password security measures, you can significantly enhance the security of your Drupal 7 site's authentication system.
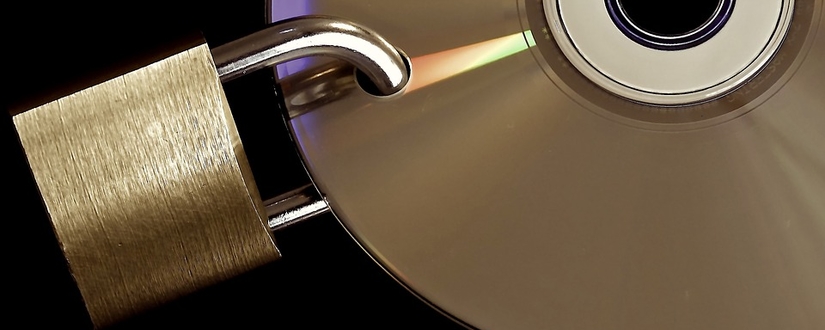