Cum să suprascrii o resursă REST din Drupal Core
Cum să suprascrii o resursă REST din Drupal Core
Uneori, proiectele ne cer să găsim soluții inovatoare. Recent, am avut nevoie să suprascriem o resursă REST din nucleul Drupal 8, și având în vedere documentația limitată disponibilă, am decis să împărtășim soluția noastră.
Premisele unei suprascieri în Drupal 8
Drupal 8 gestionează configurația într-un mod unificat, cu mai multe funcționalități stocate în nucleu decât în versiunile anterioare. Această alegere arhitecturală înseamnă că suprascrierea funcționalităților din nucleu necesită o atenție deosebită pentru a evita deteriorarea sistemului.
În timp ce Drupal 8 suportă suprascrierea globală `$config` prin `Drupal\Core\Config\ConfigFactory::get()`, suprascrierea resurselor REST necesită o abordare diferită. Nucleul Drupal 8 oferă resurse REST de bază pentru entitățile sistemului, dar operațiunile complexe necesită adesea extinderea acestor clase de bază.
Ghid pas cu pas pentru a suprascrie o resursă REST
1. Creează o nouă clasă
Mai întâi, creează o clasă care extinde clasa `EntityResource`:
namespace Drupal\my_module\Plugin\rest\resource;
use Drupal\rest\Plugin\ResourceBase;
use Drupal\rest\ResourceResponse;
use Drupal\node\Entity\Node;
/**
* @RestResource(
* id = "my_custom_node_rest_resource",
* label = @Translation("Custom Node REST Resource"),
* uri_paths = {
* "canonical" = "/api/v1/node/{node}",
* "create" = "/api/v1/node"
* }
* )
*/
class MyCustomNodeResource extends EntityResource {
....
}
2. Implementează metodele necesare
Suprascrie metodele necesare din clasa părinte:
/**
* Răspunde la cererile POST.
*
* @param array $data
* Datele cererii.
*
* @return \Drupal\rest\ResourceResponse
* Răspunsul care conține nodul.
*/
public function post($data) {
// Logica ta personalizată pentru POST aici
$response = new ResourceResponse($result, 201);
return $response;
}
/**
* Răspunde la cererile PATCH.
*
* @param \Drupal\Core\Entity\EntityInterface $entity
* Entitatea.
* @param array $data
* Datele cererii.
*
* @return \Drupal\rest\ResourceResponse
* Răspunsul.
*/
public function patch($entity, array $data) {
// Logica ta personalizată pentru PATCH aici
return new ResourceResponse($entity, 200);
}
3. Configurează setările resurselor REST
Adaugă următoarele în configurația modulului tău:
# config/install/rest.resource.my_custom_node_rest_resource.yml
langcode: en
status: true
dependencies:
module:
- my_module
- serialization
id: my_custom_node_rest_resource
plugin_id: my_custom_node_rest_resource
granularity: resource
configuration:
methods:
- GET
- POST
- PATCH
formats:
- json
authentication:
- basic_auth
4. Implementează controalele de acces
Adaugă verificarea corespunzătoare a accesului:
/**
* {@inheritdoc}
*/
public function access($operation, array $args = []) {
$entity = isset($args['entity']) ? $args['entity'] : NULL;
switch ($operation) {
case 'create':
return $this->checkCreateAccess();
case 'update':
return $this->checkUpdateAccess($entity);
default:
return parent::access($operation, $args);
}
}
```
### 5. Gestionează excepțiile
Implementează gestionarea corespunzătoare a erorilor:
```php
protected function handleException(\Exception $e) {
watchdog_exception('my_module', $e);
return new ResourceResponse([
'error' => [
'message' => $this->t('A apărut o eroare în timpul procesării cererii.'),
'code' => 500,
],
], 500);
}
Bune practici
- Menține întotdeauna compatibilitatea cu versiunile anterioare, când este posibil
- Documentează temeinic endpoint-urile tale personalizate
- Implementează controale de acces adecvate
- Adaugă gestionarea completă a erorilor
- Utilizează injecția de dependență în loc de apeluri de servicii statice
- Adaugă validarea corespunzătoare a cererilor
- Include antetele de răspuns adecvate
Testarea resursei tale personalizate
Adaugă teste PHPUnit pentru a te asigura că resursa ta funcționează conform așteptărilor:
namespace Drupal\Tests\my_module\Functional;
use Drupal\Tests\rest\Functional\ResourceTestBase;
class MyCustomNodeResourceTest extends ResourceTestBase {
// Implementarea testului
}
Capcane comune de evitat
- Negestionarea corespunzătoare a tuturor metodelor HTTP
- Uitarea actualizării configurației REST după modificări
- Gestionarea inadecvată a erorilor
- Controale de acces lipsă
- Nevalidarea datelor de intrare
- Formatarea incorectă a răspunsurilor
Urmând acești pași și aceste bune practici, poți suprascrie cu succes resursele REST din nucleul Drupal 8, menținând în același timp stabilitatea și securitatea sistemului.
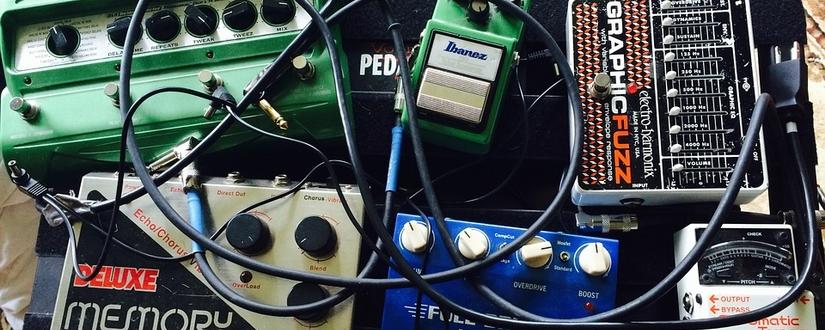