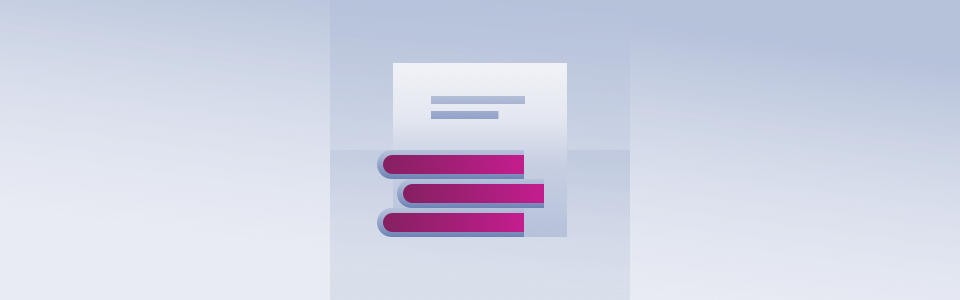
Understanding Monolithic vs. Scalable Angular Architectures
Application architecture plays a crucial role in long-term project success. While monolithic architectures might seem simpler initially, they often lead to maintenance challenges as applications grow. Let's explore why scalable architectures provide better long-term solutions for Angular applications.
A well-designed architecture should achieve three fundamental objectives:
1. Maintain or improve development velocity over time
2. Facilitate smooth implementation of new requirements
3. Preserve optimal application performance
The Challenge of Monolithic Architecture
In a monolithic architecture, applications often start with rapid development and straightforward requirement implementation. However, significant challenges emerge as the project grows:
Development velocity decreases steadily because:
- Requirements become increasingly complex to implement
- Changes in one area frequently affect other functionalities
- Developers struggle to manage the growing interconnections between components
- Regression issues become more frequent and harder to prevent
- The codebase becomes increasingly difficult to understand and maintain
Think of a monolithic architecture as building a house without proper planning for future extensions. Initially, construction moves quickly, but each addition becomes progressively more complicated and risks compromising the existing structure.
The Benefits of Scalable Architecture
A scalable architecture, while requiring more initial planning and setup, maintains consistent development velocity throughout the project lifecycle. Like Aesop's tortoise, it may start slower but maintains a steady, reliable pace that ultimately proves more effective than the rapid but unsustainable pace of a monolithic approach.
Key advantages include:
- Better project control through clear separation of concerns
- More accurate planning and estimation capabilities
- Consistent quality and performance throughout development
- Reduced technical debt
- Easier onboarding for new team members
Essential Principles for Angular Scalable Architecture
To implement a successful scalable architecture in Angular, three core principles must be followed:
1. Abstraction Layers: Create clear boundaries between different parts of your application, such as separating business logic from presentation logic. This makes the code more maintainable and testable.
2. Smart & Dumb Components:
- Smart components handle business logic and state management
- Dumb components focus solely on presentation and user interaction
This separation makes components more reusable and easier to test
3. Unidirectional Data Flow: Implement a predictable data flow pattern where:
- Data flows from parent to child components
- Events flow from child to parent components
This pattern makes state changes more predictable and easier to debug
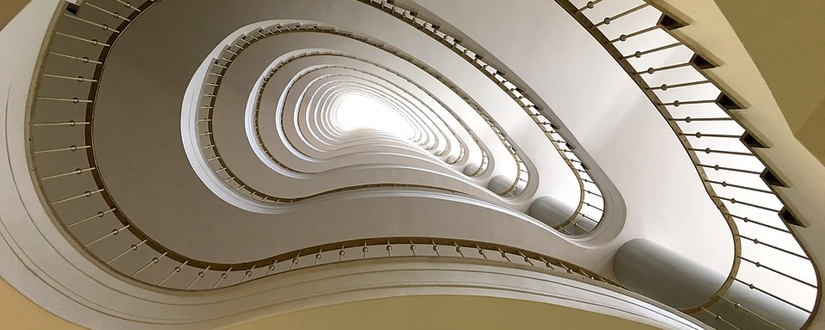
Practical Implementation
When implementing a scalable architecture:
1. Start by defining clear module boundaries
2. Create a consistent folder structure that reflects your architectural layers
3. Implement strict typing and interfaces
4. Use dependency injection effectively
5. Write comprehensive tests for each architectural layer
Remember that while the initial setup requires more time and planning, this investment pays significant dividends throughout the project's lifecycle through improved maintainability, scalability, and developer productivity.
By following these principles and patterns, you can create Angular applications that remain maintainable and performant as they grow in size and complexity. The key is to prioritize long-term sustainability over short-term development speed.
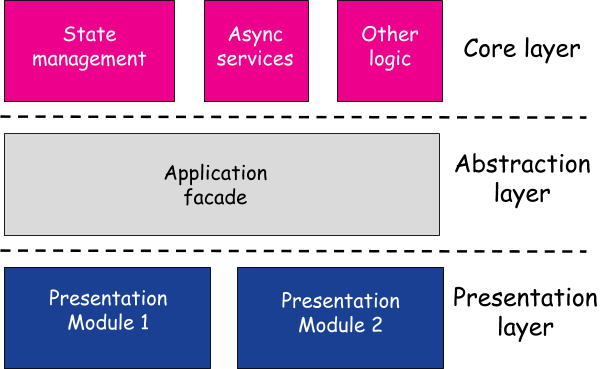