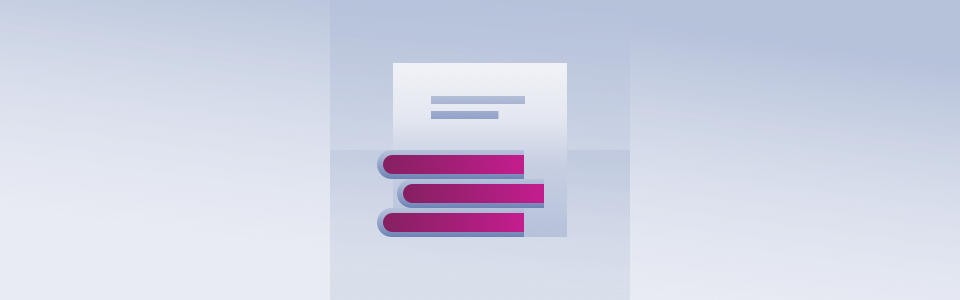
Error pages value and how to customise them in Drupal
401, 403 and 404, we all have encountered these numbers throughout our exploration of the world wide web at least once in our lives. How do you feel when these pages appear instead of what you’re looking for?
Not finding the thing you are looking for is beyond frustrating. So, these pages need to be as non-frustrating as possible, just to compensate for the initial impression and encourage users to continue browsing the website.
It goes without saying that when you start building a website, these pages come by default included in the packages. The big question is: Do we let them be, simple, unstyled or do we try making the user’s experience with them a bit more fun, design wise?
While at DrupalCamp London, I have discovered that many programmers face the challenge of making and customizing these pages. Actually, all starts with the designers that are trying to make everything as engaging and non-stressful. Then, the programmers try to customize everything and to bring it to life.
To get back to the story, in my personal experience, you shouldn’t answer with “just let them be”. These pages can have small, quirky aspects to them that make the user forget that the result is not the expected one….to forget and forgive you for your error.
Almost all my past projects have styled error page. I almost feel it like my responsibility to ask a design for these pages whenever they might be overlooked.
SO HOW DO YOU CUSTOMIZE ERROR PAGES IN DRUPAL, THEMING WISE?
Well, we have a couple of methods.
With Drupal 7 you have the page--error.tpl.php template, which you use by taking all the common regions and elements from the page.tpl.php template. Then add all your specific error page elements.
But if you want to go even deeper with the customization, change texts and have different elements for each type of error pages, then you might want a template for each of these.
You do this by adding a HOOK in your theme or module, depending on your needs.
In the past, (for Drupal 7 that is), I have used function YOURTHEME_preprocess_page
in the template.php
file that is located in the theme folder.
So a way of doing this would be:
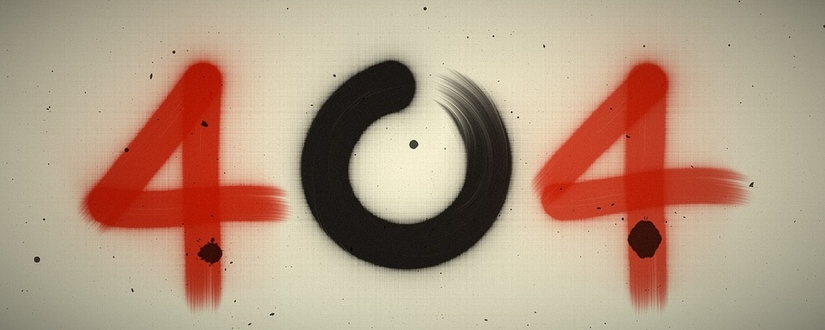
function YOURTHEME_preprocess_page(&$vars) {
$header = drupal_get_http_header('status');
if ($header == '404 Not Found') {
$vars['theme_hook_suggestions'][] = 'page__404';
}
}
Then all left to do is remember to name your template file with “--” and not “__” as you wrote it in the preprocess. So your template should be named like so: page--404.tpl.php
For Drupal 8 (my personal fav) is another story. You will have to have a different approach. Seeing that the 4xx response handling has changed, I have started using a hook_theme_suggestions_page_alter()
.
Use it in a custom module. The hook does exactly what it’s name says: it adds template suggestions for these pages. The code would look something like:
/**
* Implements hook_theme_suggestions_page_alter().
*/
function openstory_pages_theme_suggestions_page_alter(array &$suggestions, array $variables) {
$path_args = explode('/', trim(\Drupal::service('path.current')
->getPath(), '/'));
$suggestions = theme_get_suggestions($path_args, 'page');
$http_error_suggestions = [
'system.401' => 'page__401',
'system.403' => 'page__403',
'system.404' => 'page__404',
];
$route_name = \Drupal::routeMatch()->getRouteName();
if (isset($http_error_suggestions[$route_name])) {
$suggestions[] = $http_error_suggestions[$route_name];
}
}
I am sure there might be other ways to do this, but these are the methods I usually use and so far, they’ve never failed me.
So what is your favorite method of customizing an error page?